Rsa_generate_key_ex Site Www.openssl.org
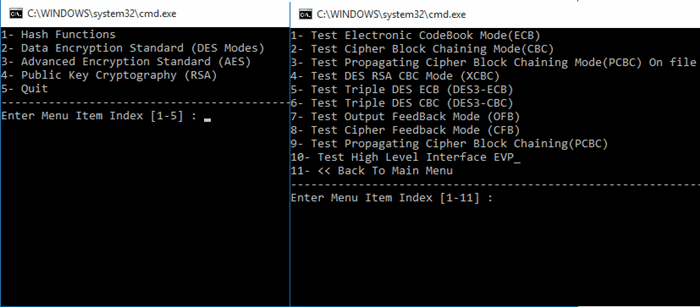
Openssl Generate Rsa Key Pair

I'm writing a small program to test an old issue on RSA. /generate-ssh-key-mac-sourcetree.html. /diablo-3-cd-key-generator-password.html. I need to access the prime factors of the modulus. So my code is int RSAKeyGen(int keySize) EVPPKEY.pkey = EVPPKEYnew. Diff -ruN openssh-7.4p1/aclocal.m4 openssh-7.4p1+x509-10.0/aclocal.m4 - openssh-7.4p1/aclocal.m4 2016-12-19 06:00000 +0200 openssh-7.4p1+x509-10.0. The branch OpenSSL102-stable has been updated via 080de69ecefe95ba05b76a0db2e253 (commit) from 9e56a32e398efe2da58c65fecea52cf4886e3c3d (commit).
prev in list next in list prev in thread next in thread List: openssl-cvs Subject: openssl-commits openssl OpenSSL-fips-202 create From: Matt Caswell Date: 2015-03-19 14:06:57 Message-ID:.62.nullmailer dev! Org Download RAW message or body The annotated tag OpenSSL-fips-202 has been created at.
Use Openssl To Generate Key Pair
- * Copyright 1995-2016 The OpenSSL Project Authors. All Rights Reserved.
- * Licensed under the OpenSSL license (the 'License'). You may not use
- * this file except in compliance with the License. You can obtain a copy
- * in the file LICENSE in the source distribution or at
- */
- /*
- * NB: these functions have been 'upgraded', the deprecated versions (which
- * are compatibility wrappers using these functions) are in rsa_depr.c. -
- */
- #include <stdio.h>
- #include 'internal/cryptlib.h'
- #include 'rsa_locl.h'
- static int rsa_builtin_keygen(RSA *rsa, int bits, BIGNUM *e_value,
- * NB: this wrapper would normally be placed in rsa_lib.c and the static
- * implementation would probably be in rsa_eay.c. Nonetheless, is kept here
- * so that we don't introduce a new linker dependency. Eg. any application
- * that wasn't previously linking object code related to key-generation won't
- * have to now just because key-generation is part of RSA_METHOD.
- int RSA_generate_key_ex(RSA *rsa, int bits, BIGNUM *e_value, BN_GENCB *cb)
- if (rsa->meth->rsa_keygen)
- return rsa->meth->rsa_keygen(rsa, bits, e_value, cb);
- return rsa_builtin_keygen(rsa, bits, e_value, cb);
- static int rsa_builtin_keygen(RSA *rsa, int bits, BIGNUM *e_value,
- {
- BIGNUM *r0 = NULL, *r1 = NULL, *r2 = NULL, *r3 = NULL, *tmp;
- BN_CTX *ctx = NULL;
- /*
- * When generating ridiculously small keys, we can get stuck
- * continually regenerating the same prime values.
- if (bits < 16) {
- RSAerr(RSA_F_RSA_BUILTIN_KEYGEN, RSA_R_KEY_SIZE_TOO_SMALL);
- }
- ctx = BN_CTX_new();
- goto err;
- r0 = BN_CTX_get(ctx);
- r2 = BN_CTX_get(ctx);
- if (r3 NULL)
- bitsq = bits - bitsp;
- /* We need the RSA components non-NULL */
- goto err;
- if (!rsa->d && ((rsa->d = BN_secure_new()) NULL))
- if (!rsa->e && ((rsa->e = BN_new()) NULL))
- if (!rsa->p && ((rsa->p = BN_secure_new()) NULL))
- if (!rsa->q && ((rsa->q = BN_secure_new()) NULL))
- if (!rsa->dmp1 && ((rsa->dmp1 = BN_secure_new()) NULL))
- if (!rsa->dmq1 && ((rsa->dmq1 = BN_secure_new()) NULL))
- if (!rsa->iqmp && ((rsa->iqmp = BN_secure_new()) NULL))
- goto err;
- /* generate p and q */
- if (!BN_generate_prime_ex(rsa->p, bitsp, 0, NULL, NULL, cb))
- if (!BN_sub(r2, rsa->p, BN_value_one()))
- if (!BN_gcd(r1, r2, rsa->e, ctx))
- if (BN_is_one(r1))
- if (!BN_GENCB_call(cb, 2, n++))
- }
- goto err;
- do {
- if (!BN_generate_prime_ex(rsa->q, bitsq, 0, NULL, NULL, cb))
- } while (BN_cmp(rsa->p, rsa->q) 0);
- goto err;
- goto err;
- break;
- goto err;
- if (!BN_GENCB_call(cb, 3, 1))
- if (BN_cmp(rsa->p, rsa->q) < 0) {
- rsa->p = rsa->q;
- }
- /* calculate n */
- goto err;
- /* calculate d */
- goto err; /* p-1 */
- goto err; /* q-1 */
- goto err; /* (p-1)(q-1) */
- BIGNUM *pr0 = BN_new();
- if (pr0 NULL)
- BN_with_flags(pr0, r0, BN_FLG_CONSTTIME);
- BN_free(pr0);
- }
- /* We MUST free pr0 before any further use of r0 */
- }
- {
- goto err;
- !BN_mod(rsa->dmp1, d, r1, ctx)
- !BN_mod(rsa->dmq1, d, r2, ctx)) {
- goto err;
- /* We MUST free d before any further use of rsa->d */
- }
- {
- goto err;
- if (!BN_mod_inverse(rsa->iqmp, rsa->q, p, ctx)) {
- goto err;
- /* We MUST free p before any further use of rsa->p */
- }
- ok = 1;
- if (ok -1) {
- ok = 0;
- if (ctx != NULL)
- BN_CTX_free(ctx);
- return ok;