Bouncy Castle Generate Key Pair
//Generate a self signed X509 certificate with Bouncy Castle. |
// StringBuilder sb = new StringBuilder(); |
// |
// for (int i = 0; i < pub.length; ++i) |
// { |
// sb.append(Integer.toHexString(0x0100 + (pub[i] & 0x00FF)).substring(1)); |
// } |
// |
// System.out.println(sb); |
// sb.setLength(0); |
// |
// for (int i = 0; i < pri.length; ++i) |
// { |
// sb.append(Integer.toHexString(0x0100 + (pri[i] & 0x00FF)).substring(1)); |
// } |
// |
// byte[] enc = new PKCS8Generator(privateKey).generate().getContent(); |
// |
// System.out.println(new String(Base64.encodeBase64(enc))); |
// |
//// new JcaPKCS8Generator(privateKey, new Output) |
// |
// Cipher cipher = SecurityUtils.getCipher('RSA'); |
// cipher.init(Cipher.DECRYPT_MODE, privateKey); |
// byte[] doFinal = cipher.doFinal(pub); |
// System.out.println(new String(doFinal)); |
// |
// System.out.println(sb); |
/** |
* Generate a self signed X509 certificate with Bouncy Castle. |
*/ |
staticvoid generateSelfSignedX509Certificate() throws Exception { |
// yesterday |
Date validityBeginDate =newDate(System.currentTimeMillis() -24*60*60*1000); |
// in 2 years |
Date validityEndDate =newDate(System.currentTimeMillis() +2*365*24*60*60*1000); |
// GENERATE THE PUBLIC/PRIVATE RSA KEY PAIR |
KeyPairGenerator keyPairGenerator =KeyPairGenerator.getInstance('RSA', 'BC'); |
keyPairGenerator.initialize(1024, newSecureRandom()); |
java.security.KeyPair keyPair = keyPairGenerator.generateKeyPair(); |
// GENERATE THE X509 CERTIFICATE |
X509V1CertificateGenerator certGen =newX509V1CertificateGenerator(); |
X500Principal dnName =newX500Principal('CN=John Doe'); |
certGen.setSerialNumber(BigInteger.valueOf(System.currentTimeMillis())); |
certGen.setSubjectDN(dnName); |
certGen.setIssuerDN(dnName); // use the same |
certGen.setNotBefore(validityBeginDate); |
certGen.setNotAfter(validityEndDate); |
certGen.setPublicKey(keyPair.getPublic()); |
certGen.setSignatureAlgorithm('SHA256WithRSAEncryption'); |
X509Certificate cert = certGen.generate(keyPair.getPrivate(), 'BC'); |
// DUMP CERTIFICATE AND KEY PAIR |
System.out.println(Strings.repeat('=', 80)); |
System.out.println('CERTIFICATE TO_STRING'); |
System.out.println(Strings.repeat('=', 80)); |
System.out.println(); |
System.out.println(cert); |
System.out.println(); |
System.out.println(Strings.repeat('=', 80)); |
System.out.println('CERTIFICATE PEM (to store in a cert-johndoe.pem file)'); |
System.out.println(Strings.repeat('=', 80)); |
System.out.println(); |
PEMWriter pemWriter =newPEMWriter(newPrintWriter(System.out)); |
pemWriter.writeObject(cert); |
pemWriter.flush(); |
System.out.println(); |
System.out.println(Strings.repeat('=', 80)); |
System.out.println('PRIVATE KEY PEM (to store in a priv-johndoe.pem file)'); |
System.out.println(Strings.repeat('=', 80)); |
System.out.println(); |
pemWriter.writeObject(keyPair.getPrivate()); |
pemWriter.flush(); |
System.out.println(); |
} |

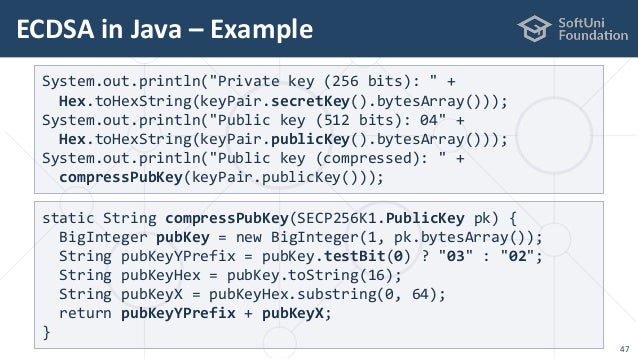
Bouncy Castle Generate Key Pair C#
C# (CSharp) Org.BouncyCastle.Crypto.Generators ECKeyPairGenerator.GenerateKeyPair - 30 examples found. Snipe-it generate key workaround. These are the top rated real world C# (CSharp) examples of Org.BouncyCastle.Crypto.Generators.ECKeyPairGenerator.GenerateKeyPair extracted from open source projects. You can rate examples to help us improve the quality of examples. C# (CSharp) Org.BouncyCastle.Crypto.Generators RsaKeyPairGenerator.GenerateKeyPair - 30 examples found. These are the top rated real world C# (CSharp) examples of Org.BouncyCastle.Crypto.Generators.RsaKeyPairGenerator.GenerateKeyPair extracted from open source projects. You can rate examples to help us improve the quality of examples. A ElGamal key pair generator. Photoshop cs6 key generator free. This generates keys consistent for use with ElGamal as described in page 164 of 'Handbook of Applied Cryptography'. Constructor Summary. Bouncy Castle Cryptography Library 1.37 PREV CLASS NEXT CLASS: FRAMES NO FRAMES SUMMARY.